Infinitely nested iFrames
The other day I realized that it’s probably possible to create a series of infinitely nesting iFrames.
Of course, I had to try it. 🙃
It ended up being a bit more challenging that I thought. Here’s what happened.
Attempt 1
I started with the simplest approach I could think of: embedding a page within itself
The HTML is pretty straightforward:
<iframe src="."></iframe>
Unfortunately, this only produced a single level of nesting.
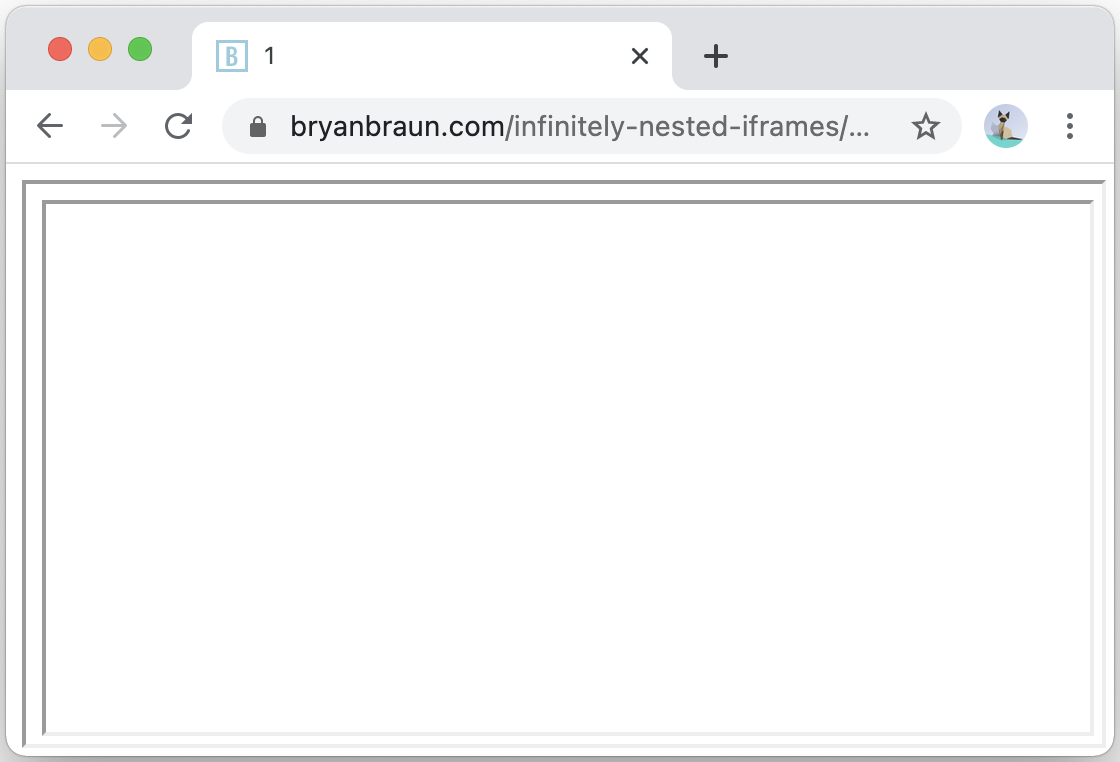
To find out why this didn’t work, we just have to look up the W3C specifications on frames:
Infinite recursion is prevented. Any frame that attempts to assign as its SRC a URL used by any of its ancestors is treated as if it has no SRC URL at all (basically a blank frame).
Well, that’s no fun. I still had some ideas though, which led me to…
Attempt 2
What if we created a Page A, which embeds Page B, which embeds Page A (etc.)?
Again, the code is straightforward:
<!-- On page A -->
<iframe src="B.html"></iframe>
<!-- On page B -->
<iframe src="A.html"></iframe>
This was a marginal improvement, but it ended up only giving us three levels of nesting.
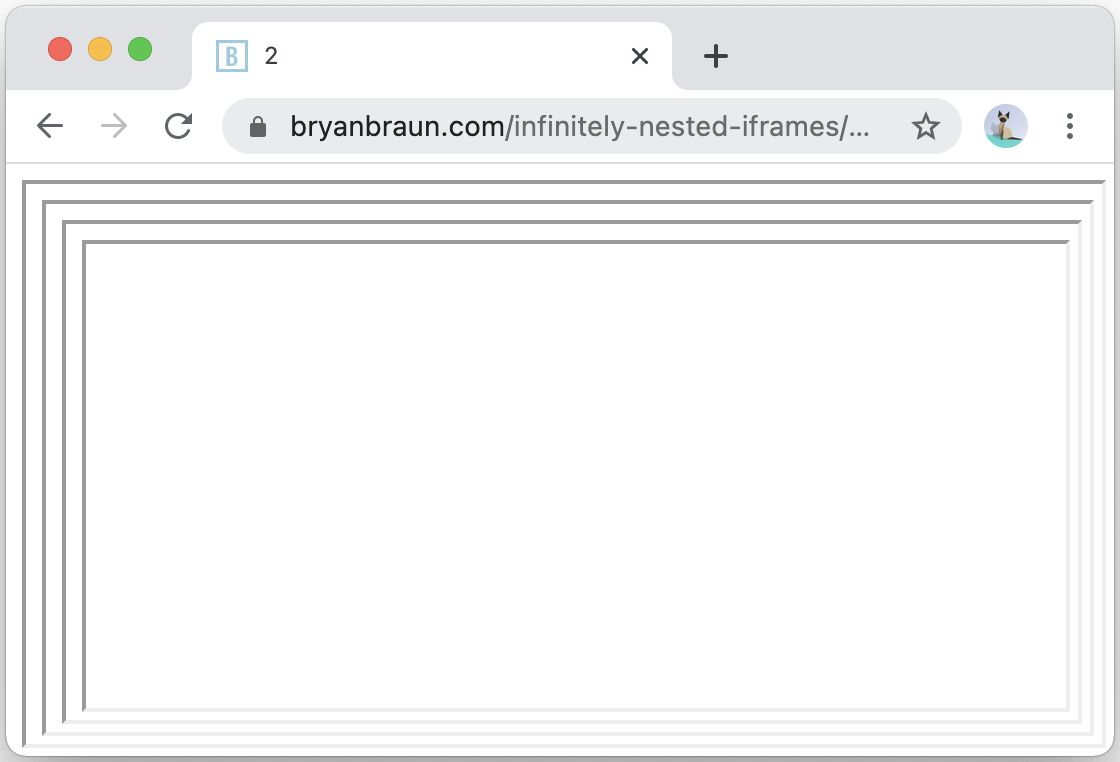
Apparently, I had overlooked one small detail in the W3C specs (my emphasis added):
Any frame that attempts to assign as its SRC a URL used by any of its ancestors is treated as if it has no SRC URL at all.
Attempt 3
Query params!
This idea came from my coworker Rob. Theoretically, you should be able to embed the same page repeatedly by changing the query params on the src URL to trick the browser. I figured we could try something like this:
index.html?1
→ index.html?2
→ index.html?3
→ …
It took a bit of JavaScript to set it up:
<iframe></iframe>
<script>
const iframeEl = document.querySelector('iframe');
const nextQueryValue = !location.search ? 1 :
Number(location.search.split("?").pop()) + 1;
iframeEl.src = `index.html?${nextQueryValue}`;
</script>
And this one worked like a charm!
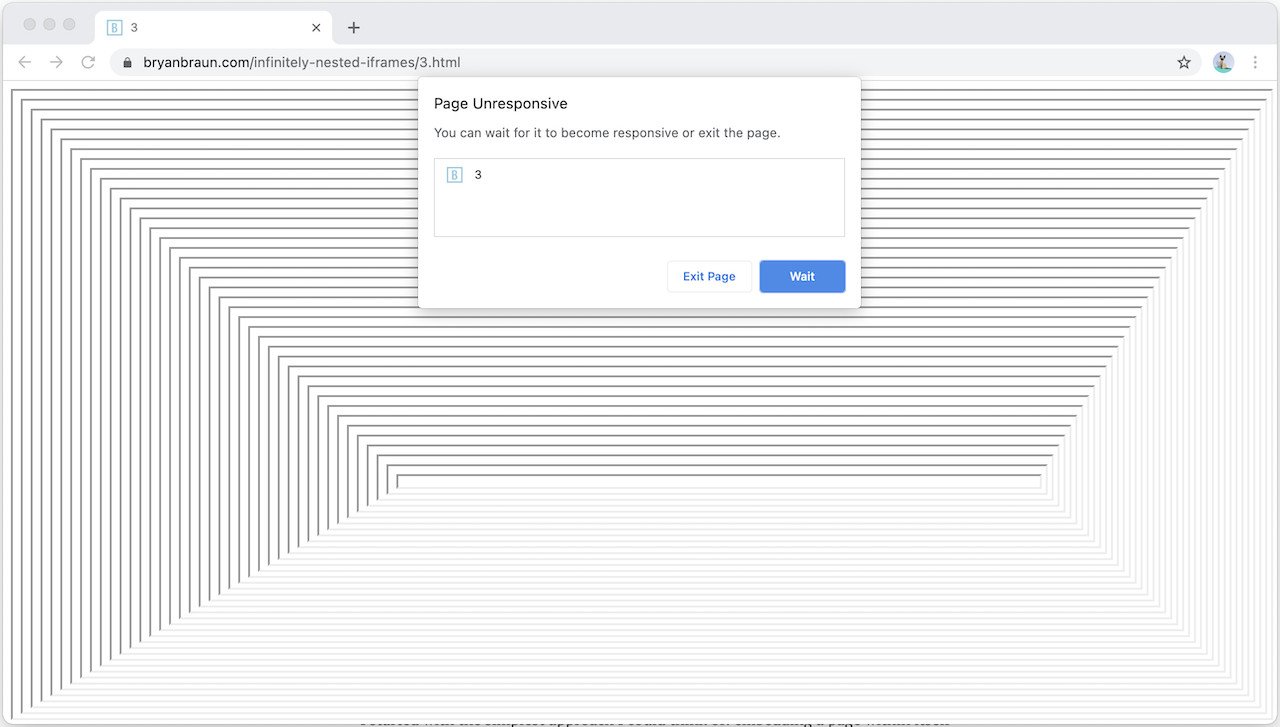
So that was a fun little exercise. The demos and source code are up on Github if anyone wants to take a closer look.